Excluding from nested models#
The exclude
option can be used to exclude fields from models that are related to our data model by using dotted
paths. For example, exclude={"a.b"}
would exclude the b
attribute of an instance nested on the a
attribute.
To demonstrate, let’s adjust our script to add an Address
model, that is related to the Person
model:
1from __future__ import annotations
2
3from dataclasses import dataclass
4
5from litestar import Litestar, get
6from litestar.dto import DataclassDTO, DTOConfig
7
8
9@dataclass
10class Address:
11 street: str
12 city: str
13 country: str
14
15
16@dataclass
17class Person:
18 name: str
19 age: int
20 email: str
21 address: Address
22
23
24class ReadDTO(DataclassDTO[Person]):
25 config = DTOConfig(exclude={"email", "address.street"})
26
27
28@get("/person/{name:str}", return_dto=ReadDTO, sync_to_thread=False)
29def get_person(name: str) -> Person:
30 # Your logic to retrieve the person goes here
31 # For demonstration purposes, a placeholder Person instance is returned
32 address = Address(street="123 Main St", city="Cityville", country="Countryland")
33 return Person(name=name, age=30, email=f"email_of_{name}@example.com", address=address)
34
35
36app = Litestar(route_handlers=[get_person])
The Address
model has three attributes, street
, city
, and country
, and we’ve added an address
attribute to the Person
model.
The ReadDTO
class has been updated to exclude the street
attribute of the nested Address
model using the
dotted path syntax "address.street"
.
Inside the handler, we create an Address
instance and assign it to the address
attribute of the Person
.
When we call our handler, we can see that the street
attribute is not included in the response:
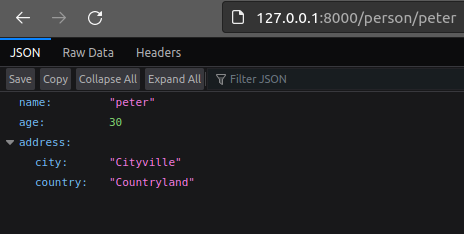