Renaming fields#
The name of a field that is used for serialization can be changed by either explicitly declaring the new name or by declaring a renaming strategy.
Explicitly renaming fields#
We can rename fields explicitly using the rename_fields
attribute. This attribute is a dictionary that maps the original field name to the new field name.
In this example, we rename the address
field to location
:
1from __future__ import annotations
2
3from dataclasses import dataclass
4
5from litestar import Litestar, get
6from litestar.dto import DataclassDTO, DTOConfig
7
8
9@dataclass
10class Address:
11 street: str
12 city: str
13 country: str
14
15
16@dataclass
17class Person:
18 name: str
19 age: int
20 email: str
21 address: Address
22 children: list[Person]
23
24
25class ReadDTO(DataclassDTO[Person]):
26 config = DTOConfig(
27 exclude={"email", "address.street", "children.0.email", "children.0.address"},
28 rename_fields={"address": "location"},
29 )
30
31
32@get("/person/{name:str}", return_dto=ReadDTO, sync_to_thread=False)
33def get_person(name: str) -> Person:
34 # Your logic to retrieve the person goes here
35 # For demonstration purposes, a placeholder Person instance is returned
36 address = Address(street="123 Main St", city="Cityville", country="Countryland")
37 child1 = Person(name="Child1", age=10, email="child1@example.com", address=address, children=[])
38 child2 = Person(name="Child2", age=8, email="child2@example.com", address=address, children=[])
39 return Person(
40 name=name,
41 age=30,
42 email=f"email_of_{name}@example.com",
43 address=address,
44 children=[child1, child2],
45 )
46
47
48app = Litestar(route_handlers=[get_person])
Notice how the address
field is renamed to location
.
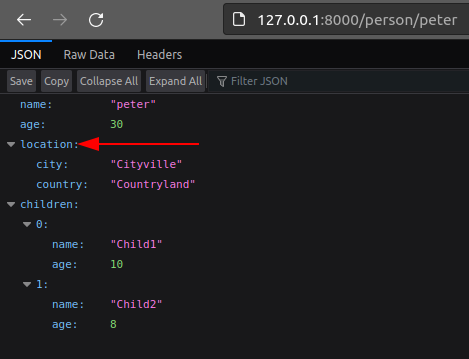
Field renaming strategies#
Instead of explicitly renaming fields, we can also use a field renaming strategy.
The field renaming strategy is specified using the
rename_strategy
config.
Litestar supports the following strategies:
lower
: Converts the field name to lowercaseupper
: Converts the field name to uppercasecamel
: Converts the field name to camel casepascal
: Converts the field name to pascal case
Note
You can also define your own strategies by passing a callable that receives the field name, and returns the new
field name to the rename_strategy
config.
Let’s modify our example to use the upper
strategy:
1from __future__ import annotations
2
3from dataclasses import dataclass
4
5from litestar import Litestar, get
6from litestar.dto import DataclassDTO, DTOConfig
7
8
9@dataclass
10class Address:
11 street: str
12 city: str
13 country: str
14
15
16@dataclass
17class Person:
18 name: str
19 age: int
20 email: str
21 address: Address
22 children: list[Person]
23
24
25class ReadDTO(DataclassDTO[Person]):
26 config = DTOConfig(
27 exclude={"email", "address.street", "children.0.email", "children.0.address"},
28 rename_strategy="upper",
29 )
30
31
32@get("/person/{name:str}", return_dto=ReadDTO, sync_to_thread=False)
33def get_person(name: str) -> Person:
34 # Your logic to retrieve the person goes here
35 # For demonstration purposes, a placeholder Person instance is returned
36 address = Address(street="123 Main St", city="Cityville", country="Countryland")
37 child1 = Person(name="Child1", age=10, email="child1@example.com", address=address, children=[])
38 child2 = Person(name="Child2", age=8, email="child2@example.com", address=address, children=[])
39 return Person(
40 name=name,
41 age=30,
42 email=f"email_of_{name}@example.com",
43 address=address,
44 children=[child1, child2],
45 )
46
47
48app = Litestar(route_handlers=[get_person])
And the result:
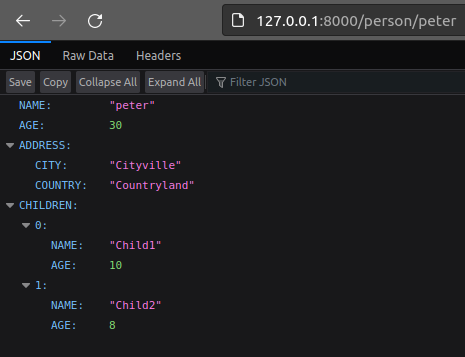