CLI#
Litestar provides a convenient command line interface (CLI) for running and managing Litestar applications. The CLI is powered by click, rich, and rich-click.
Enabling all CLI features#
The CLI and its hard dependencies are included by default. However, if you want to run your application
(using litestar run
) or beautify the Typescript generated by the litestar schema typescript
command, you’ll need uvicorn
and jsbeautifier
. They can be installed independently, but we
recommend installing the standard
group which conveniently bundles commonly used optional dependencies.
pip install litestar[standard]
Once you have installed standard
, you’ll have access to the litestar run
command.
Autodiscovery#
Litestar offers autodiscovery of applications and application factories placed within the canonical modules named
either app
or application
. These modules can be individual files or directories. Within these modules or their
submodules, the CLI will detect any instances of Litestar
, callables named create_app
, or
callables annotated to return a Litestar
instance.
The autodiscovery follows these lookup locations in order:
app.py
app/__init__.py
Submodules of
app
application.py
application/__init__.py
Submodules of
application
Within these locations, Litestar CLI looks for:
Commands#
litestar#
The main entrypoint to the Litestar CLI is the litestar
command.
If you don’t pass the --app
flag, the application will be automatically discovered, as explained in
Autodiscovery.
Options#
Flag |
Environment variable |
Description |
---|---|---|
|
|
|
|
N/A |
Look for the app in the specified directory by adding it to the PYTHONPATH. Defaults to the current working directory. |
version#
Prints the currently installed version of Litestar.
Options#
Name |
Description |
---|---|
|
Include only |
run#
The run
command executes a Litestar application using uvicorn.
litestar run
Caution
This feature is intended for development purposes only and should not be used to deploy production applications.
Changed in version 2.8.0: CLI options take precedence over environment variables!
Options#
Flag |
Environment variable |
Description |
---|---|---|
|
|
Reload the application when files in its directory are changed |
|
|
Specify directories to watch for reload. |
|
|
Specify glob patterns for files to include when watching for reload. |
|
|
Specify glob patterns for files to exclude when watching for reload. |
|
|
Bind the server to this port [default: 8000] |
|
|
Changed in version 2.8: The number of concurrent web workers to start [default: 1] |
|
|
Bind the server to this host [default: 127.0.0.1] |
|
|
Bind to a socket from this file descriptor. |
|
|
Bind to a UNIX domain socket. |
|
|
Run the application in debug mode |
|
|
Drop into the Python debugger when an exception occurs |
|
|
Path to a SSL certificate file |
|
|
Path to the private key to the SSL certificate |
|
|
If the SSL certificate and key are not found, generate a self-signed certificate |
–reload-dir#
The --reload-dir
flag allows you to specify directories to watch for changes. If you specify this flag, the --reload
flag is implied. You can specify multiple directories by passing the flag multiple times:
litestar run --reload-dir=. --reload-dir=../other-library/src
To set multiple directories via an environment variable, use a comma-separated list:
LITESTAR_RELOAD_DIRS=.,../other-library/src
–reload-include#
The --reload-include
flag allows you to specify glob patterns to include when watching for file changes. If you specify this flag, the --reload
flag is implied. Furthermore, .py
files are included implicitly by default.
You can specify multiple glob patterns by passing the flag multiple times:
litestar run --reload-include="*.rst" --reload-include="*.yml"
To set multiple directories via an environment variable, use a comma-separated list:
LITESTAR_RELOAD_INCLUDES=*.rst,*.yml
–reload-exclude#
The --reload-exclude
flag allows you to specify glob patterns to exclude when watching for file changes. If you specify this flag, the --reload
flag is implied.
You can specify multiple glob patterns by passing the flag multiple times:
litestar run --reload-exclude="*.py" --reload-exclude="*.yml"
To set multiple directories via an environment variable, use a comma-separated list:
LITESTAR_RELOAD_EXCLUDES=*.py,*.yml
SSL#
You can pass paths to an SSL certificate and it’s private key to run the server using the HTTPS protocol:
litestar run --ssl-certfile=certs/cert.pem --ssl-keyfile=certs/key.pem
Both flags must be provided and both files must exist. These are then passed to uvicorn
.
You can also use the --create-self-signed-cert
flag:
litestar run --ssl-certfile=certs/cert.pem --ssl-keyfile=certs/key.pem --create-self-signed-cert
This way, if the given files don’t exist, a self-signed certificate and a passwordless key will be generated. If the files are found, they will be reused.
info#
The info
command displays useful information about the selected application and its configuration.
litestar info
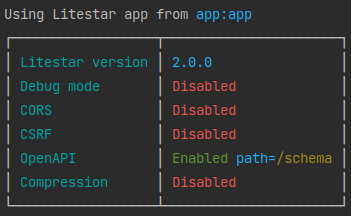
routes#
The routes
command displays a tree view of the routing table.
litestar routes
Options#
Flag |
Description |
---|---|
|
Include default auto generated openAPI schema routes |
|
Exclude endpoints from query with given regex patterns. Multiple excludes allowed. e.g., |
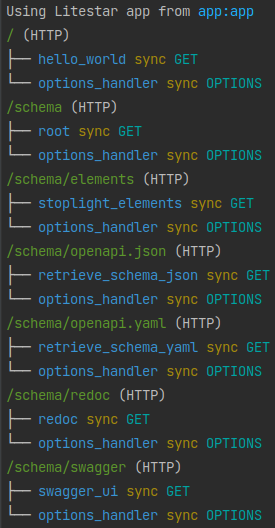
sessions#
This command and its subcommands provide management utilities for server-side session backends.
delete#
The delete
subcommand deletes a specific session from the backend.
litestar sessions delete cc3debc7-1ab6-4dc8-a220-91934a473717
clear#
The clear subcommand is used to remove all sessions from the backend.
litestar sessions clear
openapi#
This command provides utilities to generate OpenAPI schemas and TypeScript types.
schema#
The schema subcommand generates OpenAPI specifications from the Litestar application and serializes them as either JSON or YAML. The serialization format depends on the filename, which is by default openapi_schema.json. You can specify a different filename using the –output flag. For example:
litestar schema openapi --output my-specs.yml
typescript#
The typescript subcommand generates TypeScript definitions from the Litestar application’s OpenAPI specifications. For example:
litestar schema typescript
By default, this command outputs a file called api-specs.ts. You can change this using the –output option:
litestar schema typescript --output my-types.ts
You can also specify the top-level TypeScript namespace that will be created, which is API by default:
export namespace API {
// ...
}
To do this, use the –namespace option:
litestar schema typescript --namespace MyNamespace
This will result in:
export namespace MyNamespace {
// ...
}
Extending the CLI#
Litestar’s CLI is built with click and can be
extended by making use of
entry points,
or by creating a plugin that conforms to the
CLIPluginProtocol
.
Using entry points#
Entry points for the CLI can be added under the litestar.commands
group. These
entries should point to a click.Command
or click.Group
:
from setuptools import setup
setup(
name="my-litestar-plugin",
...,
entry_points={
"litestar.commands": ["my_command=my_litestar_plugin.cli:main"],
},
)
Using a plugin#
A plugin extending the CLI can be created using the
CLIPluginProtocol
. Its
on_cli_init()
will be called during the
initialization of the CLI, and receive the root click.Group
as its first
argument, which can then be used to add or override commands:
from litestar import Litestar
from litestar.plugins import CLIPluginProtocol
from click import Group
class CLIPlugin(CLIPluginProtocol):
def on_cli_init(self, cli: Group) -> None:
@cli.command()
def is_debug_mode(app: Litestar):
print(app.debug)
app = Litestar(plugins=[CLIPlugin()])
Accessing the app instance#
When extending the Litestar CLI, you will most likely need access to the loaded Litestar
instance.
You can achieve this by adding the special app
parameter to your CLI functions. This will cause the
Litestar
instance to be injected into the function whenever it is called from a click-context.
import click
from litestar import Litestar
@click.command()
def my_command(app: Litestar) -> None: ...
CLI Reference#
For more information, visit the Litestar CLI Click API Reference.