Receiving data#
So far, we’ve only returned data to the client, however, this is only half of the story. We also need to be able to control the data that we receive from the client.
Here’s the code we’ll use to start:
1from __future__ import annotations
2
3from dataclasses import dataclass
4
5from litestar import Litestar, post
6from litestar.dto import DataclassDTO, DTOConfig
7
8
9@dataclass
10class Person:
11 name: str
12 age: int
13 email: str
14
15
16class ReadDTO(DataclassDTO[Person]):
17 config = DTOConfig(exclude={"email"})
18
19
20@post("/person", return_dto=ReadDTO, sync_to_thread=False)
21def create_person(data: Person) -> Person:
22 # Logic for persisting the person goes here
23 return data
24
25
26app = Litestar(route_handlers=[create_person])
To simplify our demonstration, we’ve reduced our data model back down to a single Person
class, with name
age
and email
attributes.
As before, ReadDTO
is configured for the handler, and excludes the email
attribute from return payloads.
Our handler is now a @post()
handler, that is annotated to both accept and return an
instance of Person
.
Litestar can natively decode request payloads into Python dataclasses
, so we don’t
need a DTO defined for the inbound data for this script to work.
Now that we need to send data to the server to test our program, you can use a tool like Postman or Posting. Here’s an example of a request/response payload:
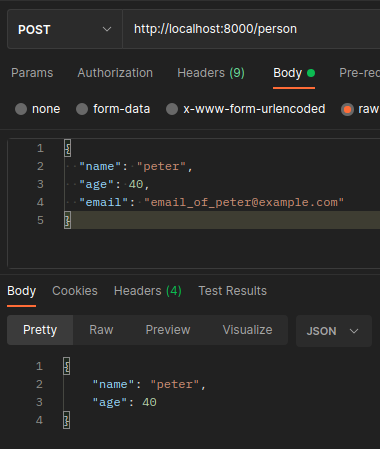