Data Transfer Object Tutorial#
Who is this tutorial for?
This tutorial is intended to familiarize you with the basic concepts of Litestar’s Data Transfer Objects (DTOs). It is assumed that you are already familiar with Litestar and fundamental concepts such as route handlers. If not, it is recommended to first follow the Developing a basic TODO application tutorial.
In this tutorial, we will walk through the process of modelling a simple data structure, and demonstrate how Litestar’s DTO factories can be used to help us build flexible applications. Lets get started!
app.py
#from __future__ import annotations
from dataclasses import dataclass
from litestar import Litestar, get
@dataclass
class Person:
name: str
age: int
email: str
@get("/person/{name:str}", sync_to_thread=False)
def get_person(name: str) -> Person:
# Your logic to retrieve the person goes here
# For demonstration purposes, a placeholder Person instance is returned
return Person(name=name, age=30, email=f"email_of_{name}@example.com")
app = Litestar(route_handlers=[get_person])
In this script, we define a data model, a route handler and an application instance.
Our data model is a Python dataclass
called Person
which has three attributes:
name
, age
, and email
.
The function called get_person
that is decorated with @get()
is a route handler
with path /person/{name:str}
, that serves GET
requests. In the path, {name:str}
represents a path parameter called name
with a string type. The route handler
receives the name from the path parameter and returns a Person
object.
Finally, we create an application instance and register the route handler with it.
In Litestar, this pattern works “out-of-the-box” - that is, returning dataclass
instances from handlers is natively supported. Litestar will take that dataclass instance, and transform it into
bytes
that can be sent over the network.
Lets run it and see for ourselves!
Save the above script as app.py
, run it using the litestar run
command, and then visit
http://localhost:8000/person/peter in your browser. You should see the
following:
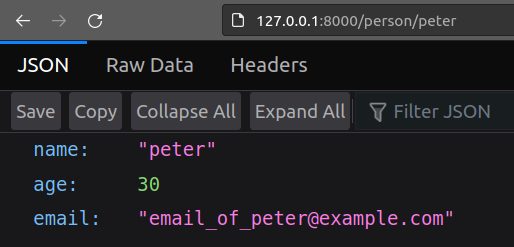
However, real-world applications are rarely this simple. What if we want to restrict the information about users that we expose after they have been created? For example, we may want to hide the user’s email address from the response. This is where Data Transfer Objects come in.