CLI#
Starlite optionally provides a simple command line interface, for running and managing Starlite applications, powered by click and rich.
Enabling the CLI#
Dependencies for the CLI are not included by default, to keep the packages needed to install
Starlite to a minimum. To enable the CLI, Starlite has to be installed with the cli
or standard
extra:
pip install starlite[cli]
pip install starlite[standard]
After installing any of these two, the starlite
command will be available as the entrypoint
to the CLI.
Autodiscovery#
Starlite will automatically discover Starlite applications and application factories in certain places:
app.py
application.py
asgi.py
app/__init__.py
If any of these files contains an instance of the Starlite
class, a function named
create_app
, or a function annotated as returning a Starlite
instance, the CLI will pick it up.
Commands#
Starlite#
The starlite
command is the main entrypoint to the CLI. If the --app
flag is not passed,
the app will be automatically discovered as described in the section above
Options#
Flag |
Environment variable |
Description |
---|---|---|
|
|
|
Run#
The run
command runs a Starlite application using uvicorn.
starlite run
Caution
This feature is intended for development purposes only and should not be used to deploy production applications
Options#
Flag | Environment variable | Description |
|||
---|---|---|---|
|
|
||
|
|
Bind the the server to this port [default: 8000] |
|
|
|
Bind the server to this host [default: 127.0.0.1] |
|
|
|
Run the application in debug mode |
|
|
|
Run the application in debug mode |
Info#
The info
command displays useful information about the selected application and its configuration
starlite info
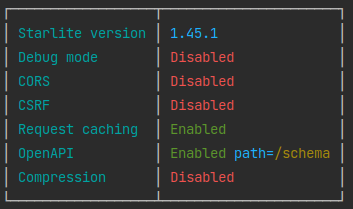
Routes#
The routes
command displays a tree view of the routing table
starlite routes
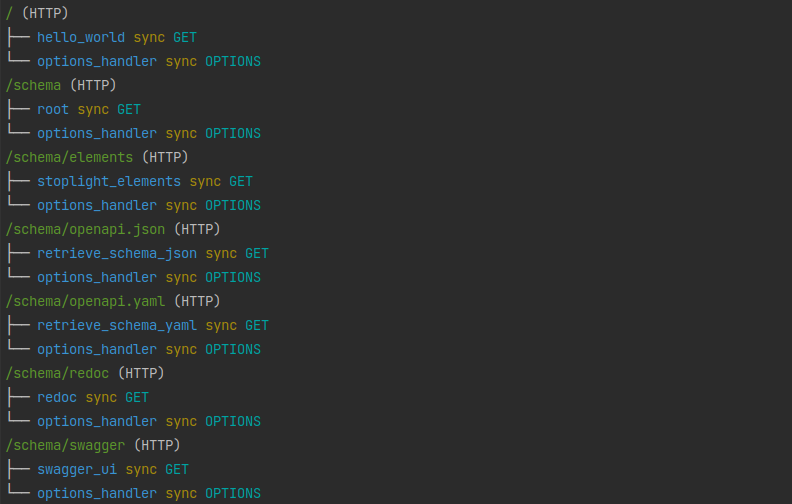
Sessions#
This command and its subcommands provide management utilities for server-side session backends.
Delete#
The delete
subcommand deletes a specific session from the backend.
starlite sessions delete cc3debc7-1ab6-4dc8-a220-91934a473717
Clear#
The clear
subcommand clears all sessions from the backend.
starlite sessions clear
OpenAPI#
This command provides utilities to generate OpenAPI schema and TypeScript types.
Schema#
The schema
subcommand generates OpenAPI specs from the Starlite application, serializing these as either JSON or YAML.
The serialization format depends on the filename, which is by default openapi_schema.json
. You can specify a different
filename using the --output
flag. For example:
starlite openapi schema --output my-specs.yaml
TypeScript#
The typescript
subcommand generates TypeScript definitions from the Starlite application’s OpenAPI specs. For example:
starlite openapi typescript
By default, this command will output a file called api-specs.ts
. You can change this using the --output
option:
starlite openapi typescript --output my-types.ts
You can also specify the top level TypeScript namespace that will be created, which by default will be called API:
export namespace API {
// ...
}
To do this use the --namespace
option:
starlite openapi typescript --namespace MyNamespace
Which will result in:
export namespace MyNamespace {
// ...
}
Extending the CLI#
Starlite’s CLI is built with click, and can be easily extended.
All that’s needed to add subcommands under the starlite
command is adding an
entry point, pointing
to a click.Command
or click.Group
, under the
starlite.commands
group.
from setuptools import setup
setup(
name="my-starlite-plugin",
...,
entry_points={
"starlite.commands": ["my_command=my_starlite_plugin.cli:main"],
},
)
[tool.poetry.plugins."starlite.commands"]
my_command = "my_starlite_plugin.cli:main"
Accessing the app instance#
When extending the Starlite CLI, you most likely need access to the loaded Starlite
instance.
This can be achieved by adding the special app
parameter to your CLI functions. This will cause
Starlite
instance to be injected into the function whenever it is being called from a click-context.
import click
from starlite import Starlite
@click.command()
def my_command(app: Starlite) -> None:
...