Updating instances#
In this section we’ll see how to update existing instances using DTOData
.
PUT handlers#
PUT requests are characterized by requiring the full data model to be submitted for update.
1from __future__ import annotations
2
3from dataclasses import dataclass
4
5from litestar import Litestar, put
6from litestar.dto import DataclassDTO, DTOConfig, DTOData
7
8
9@dataclass
10class Person:
11 name: str
12 age: int
13 email: str
14 id: int
15
16
17class ReadDTO(DataclassDTO[Person]):
18 config = DTOConfig(exclude={"email"})
19
20
21class WriteDTO(DataclassDTO[Person]):
22 config = DTOConfig(exclude={"id"})
23
24
25@put("/person/{person_id:int}", dto=WriteDTO, return_dto=ReadDTO, sync_to_thread=False)
26def update_person(person_id: int, data: DTOData[Person]) -> Person:
27 # Usually the Person would be retrieved from a database
28 person = Person(id=person_id, name="John", age=50, email="email_of_john@example.com")
29 return data.update_instance(person)
30
31
32app = Litestar(route_handlers=[update_person])
This script defines a PUT
handler with path /person/{person_id:int}
that includes a route parameter,
person_id
to specify which person should be updated.
In the handler, we create an instance of Person
, simulating a database lookup, and then pass it to the
DTOData.update_instance()
method, which returns the same instance
after modifying it with the submitted data.
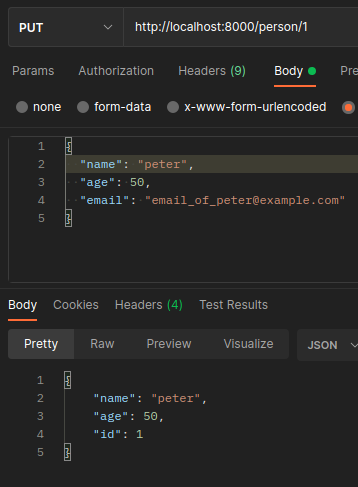
PATCH handlers#
PATCH requests are characterized by allowing any subset of the properties of the data model to be submitted for update. This is in contrast to PUT requests, which require the entire data model to be submitted.
1from __future__ import annotations
2
3from dataclasses import dataclass
4
5from litestar import Litestar, patch
6from litestar.dto import DataclassDTO, DTOConfig, DTOData
7
8
9@dataclass
10class Person:
11 name: str
12 age: int
13 email: str
14 id: int
15
16
17class ReadDTO(DataclassDTO[Person]):
18 config = DTOConfig(exclude={"email"})
19
20
21class PatchDTO(DataclassDTO[Person]):
22 config = DTOConfig(exclude={"id"}, partial=True)
23
24
25@patch("/person/{person_id:int}", dto=PatchDTO, return_dto=ReadDTO, sync_to_thread=False)
26def update_person(person_id: int, data: DTOData[Person]) -> Person:
27 # Usually the Person would be retrieved from a database
28 person = Person(id=person_id, name="John", age=50, email="email_of_john@example.com")
29 return data.update_instance(person)
30
31
32app = Litestar(route_handlers=[update_person])
In this latest update, the handler has been changed to a @patch()
handler.
This script introduces the PatchDTO
class that has a similar configuration to WriteDTO
, with the id
field
excluded, but it also sets partial=True
. This setting allows for
partial updates of the resource.
And here’s a demonstration of use:
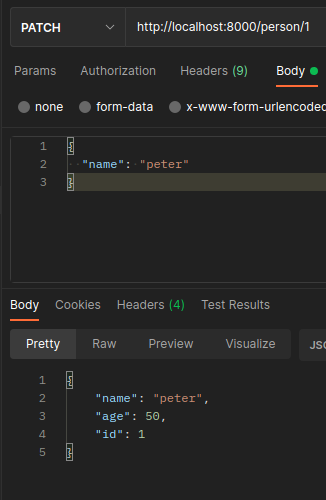